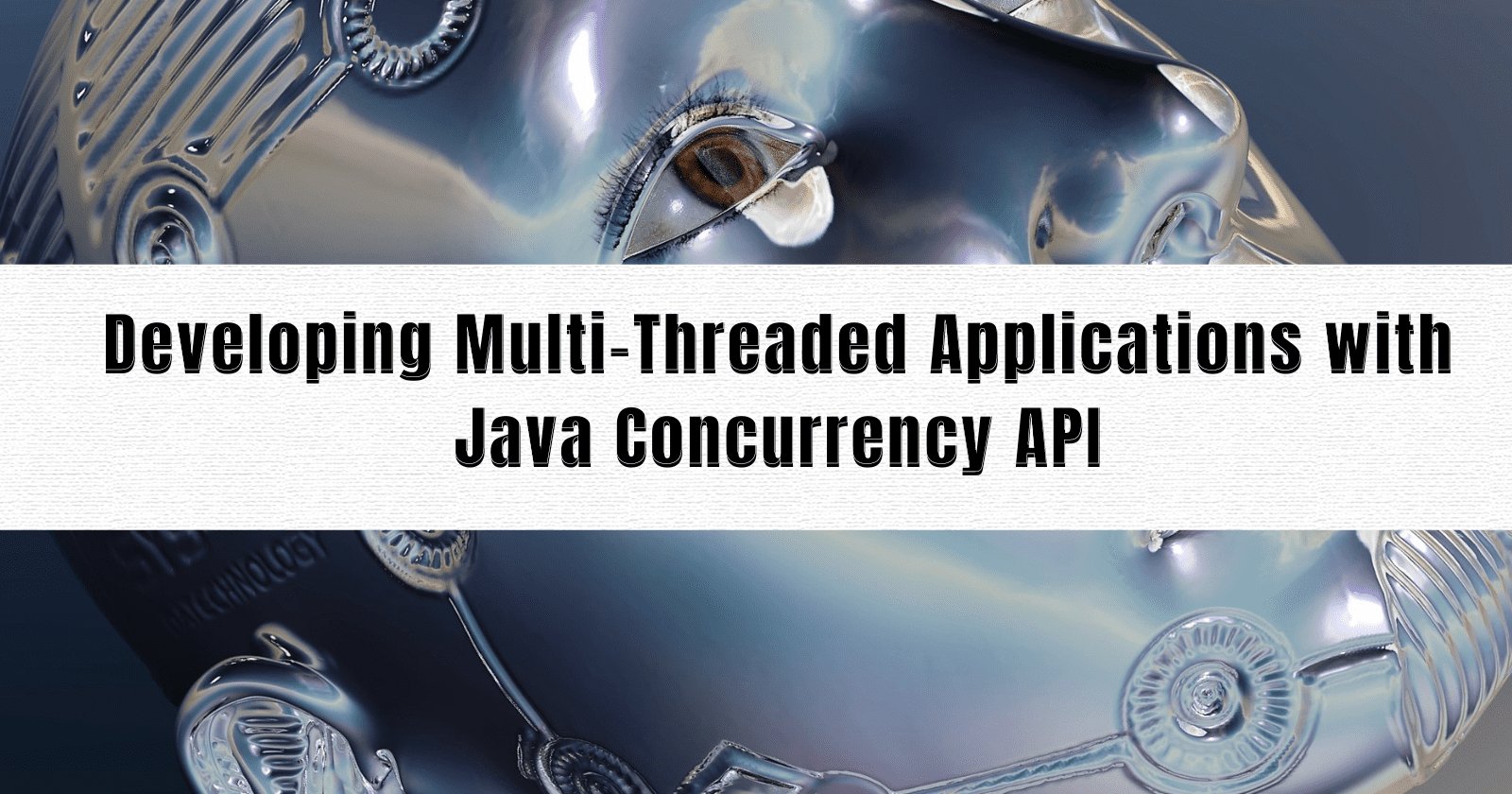
Introduction
Java Concurrency API is a set of Java packages and classes developed to create multi-threaded applications. It was introduced in Java 5 and is aimed to make writing easier for concurrent and parallel code in Java. The Java Concurrency API offers classes and utilities that allow developers to create and manage threads, synchronize access to shared resources, and perform parallel algorithms. It includes features such as thread pools, locks, condition variables, and atomic variables, which help to manage the synchronization and coordination of threads. However, implementing multi-threaded systems can be complex and error-prone, and using the Java Concurrency API requires a good understanding of its features and best practices. This article aims to provide an introduction to the Java Concurrency API and its core features to help developers in getting started with writing concurrent and parallel programs in Java.
Understanding Threads in Java
Threads are a fundamental concept in Java concurrency, and understanding how they work is required for designing multi-threaded applications with the Java Concurrency API.
A thread can be thought of as an independent path of execution within a Java application. Each thread has its own stack and program counter and can run code concurrently with other threads. Java applications usually start with a single thread of execution known as the main thread, which is responsible for executing the main() method.
In Java, developers can either extend the Thread class or implement the Runnable interface to create a new thread. The Thread class includes a run() method that serves as the thread’s entry point and can be overridden by the developer to define the thread’s behavior. Alternatively, the Runnable interface provides a single run() method that the developer can implement and send as a parameter to the Thread constructor.
After creating a thread, it can be started with the start() method. This method initiates the thread execution and invokes the run() method. When the run() method completes, the thread terminates.
Java threads can be paused, resumed, and terminated using methods such as sleep(), wait(), and interrupt(). Also, Threads can communicate with one another and synchronize access to shared resources by using synchronization structures such as synchronized blocks, locks, and condition variables.
It’s important to remember that multi-threaded programming can be complex and prone to errors like race conditions and deadlocks. It’s essential to follow best practices when developing multi-threaded applications in Java, such as avoiding the shared mutable state, using immutable objects, and minimizing synchronization.
Concept of Synchronization and Thread Safety
Synchronization
- Synchronization is the process of controlling access to shared resources in a multi-threaded environment.
- Synchronization is implemented using constructs like synchronized methods, synchronized statements, and locks.
- When a method or statement is synchronized, only one thread can access it at a time. All subsequent threads that attempt to access the same method or statement are blocked until the initial thread releases the lock.
- Synchronization is required to avoid race conditions, which occur when two or more threads access a shared resource at the same time, resulting in data corruption.
- For example, consider a scenario where two threads try to update a shared counter variable simultaneously. If both threads read the counter value, increment it, and write it back, the result may be incorrect because each thread is unaware of the other’s update.
Thread Safety
- Thread safety refers to the ability of a program to function correctly in a multi-threaded environment, without data corruption, race conditions, or other concurrency-related bugs.
- Thread safety is important for shared data structures, such as lists, maps, and sets, that can be accessed concurrently by multiple threads.
- Java includes various thread-safe collection classes, such as ConcurrentHashMap and CopyOnWriteArrayList, that ensure safe and efficient concurrent access.
Developers must pay careful attention to synchronization and thread safety when developing multi-threaded applications, as incorrect synchronization can lead to deadlocks, livelocks, and other concurrency-related issues. Best practices for synchronization and thread safety include minimizing shared mutable state, using immutable objects, and using high-level abstractions like the java.util.concurrent package.
Creating and Managing Threads in Java
Creating and managing threads in Java is a necessary step for developing multi-threaded applications that use the Java Concurrency API. Here are some examples to explain the process:
- Java threads can be created by extending the Thread class or implementing the Runnable interface.
- Extending the Thread class involves overriding the run() method, which determines the thread’s behavior. Implementing the Runnable interface necessitates implementing the run() method and passing it as a parameter to the Thread constructor. This also helps in constructor overloading.
- After creating a thread, it can be started using the start() method. This initiates the thread’s execution and calls the run() method.
- The thread can also be paused by using the wait() method, which causes it to wait until another thread notifies it that it is ready to restart.
- Java threads can communicate with one another and use synchronization constructs such as synchronized blocks, locks, and condition variables to synchronize access to shared resources.
- Interrupting Java threads is also possible with the interrupt() method, which sets a flag indicating that the thread should stop executing.
- The Java Concurrency API includes many high-level abstractions for thread management, such as the Executor framework, which simplifies thread pool management and task execution.
Developing multi-threaded Java applications requires paying careful attention to thread safety and synchronization, as inappropriate use of concurrency constructs can result in subtle and hard-to-debug errors.
Thread Pools and Executors in Java
Thread Pool: A thread pool is a group of threads that can be reused to perform multiple tasks concurrently. A thread pool maintains a pool of threads that can be used to execute tasks rather than creating and destroying threads every time a job needs to be executed. The thread pool allocates tasks to available threads in the pool, reducing the overhead of creating and destroying threads.
Executor: An executor is an object that manages the execution of tasks in a thread pool. Executors in Java Concurrency API abstract thread creation and management and provide a high-level API for executing tasks in a multi-threaded environment.
Benefits of using Thread Pool and Executor
- Efficient use of resources: Thread pools and executors allow efficient use of system resources by reusing threads instead of creating and destroying threads repeatedly.
- Improved performance: Thread pools and executors can improve application performance by executing tasks concurrently, reducing the time taken to complete the tasks.
- Simplify concurrency management: Thread pools and executors abstract the complexity of thread management, making it easier to write concurrent code that is both thread-safe and scalable.
How to use Thread Pools and Executors in Java?
The Java Concurrency API provides many Executor interface implementations for creating and managing thread pools. Let’s look at some of the most commonly used thread pools and executors.
- Fixed Thread Pool
A fixed thread pool is one that has a fixed number of threads. Once the thread pool is created, the number of threads in the pool remains constant. You can create a fixed thread pool using the following code:
ExecutorService executor =
Executors.newFixedThreadPool(5);
- Cached Thread Pool
A cached thread pool creates new threads as needed and then releases them when they become idle. You can create a Cached thread pool using the following code:
ExecutorService executor =
Executors.newCachedThreadPool();
The above code creates a cached thread pool with an unbounded number of threads.
- Scheduled Thread Pool
A scheduled thread pool is a thread pool that can execute tasks periodically or after a delay. You can create a Scheduled thread pool using the following code:
ScheduledExecutorService executor =
Executors.newScheduledThreadPool(5);
The above code creates a scheduled thread pool of five threads.
- Single Thread Executor
A single thread executor is a thread pool with only one thread. You can create a Single thread executor using the following code:
ExecutorService executor =
Executors.newSingleThreadExecutor();
The above code creates a single thread executor.
Once a thread pool is created, you can submit tasks to it using the execute() method of the Executor interface. The execute() method accepts a Runnable object as a parameter and executes it on one of the threads in the thread pool. Here is an example:
executor.execute(new Runnable() {
public void run() {
// Task logic here
}
});
In the above code, we submit a new Runnable task to the thread pool using the execute() method.
Best Practices for Developing Multi-Threaded Applications in Java
Developing multi-threaded applications in Java can be challenging due to the complexity involved in managing threads and ensuring thread safety. Here are some best practices for writing efficient, scalable, and thread-safe multi-threaded applications in Java.
Use Thread Pools and Executors
Thread pools and executors help in the effective management and reuse of threads. They abstract the complexity of thread management and provide a high-level API for executing tasks in a multi-threaded environment. Using thread pools and executors can help you write scalable and efficient code.
Avoid Shared Mutable State
A shared mutable state occurs when two or more threads share a data structure, and at least one thread can modify it. Shared mutable state can lead to race conditions, deadlocks, and other concurrency issues. To overcome these issues, use immutable data structures or synchronize access to shared mutable states.
Use Atomic Variables
Atomic variables are thread-safe variables that can be updated atomically. They provide a way to avoid race conditions when multiple threads access the same variable. Atomic variables are available in Java Concurrency API and can be used to ensure thread safety.
Use Volatile Variables
Volatile variables are variables that are always read and written from the main memory rather than the local cache of a thread. Volatile variables allow you to ensure that the value of a variable is visible to all threads. Using volatile variables can help you avoid concurrency issues such as race conditions.
Use Synchronization and Locks
Synchronization and locks provide a way to ensure that only one thread can access a critical section of code at a time. You should use synchronization and locks whenever you need to access a shared mutable state.
Use Thread-Safe Classes
The Java Concurrency API includes various thread-safe classes for writing thread-safe code. For example, the ConcurrentHashMap class is a thread-safe implementation of the Map interface. Using thread-safe classes allows you to build thread-safe code without worrying about underlying concurrency issues.
Use Immutable Classes
Immutable classes are classes whose instances cannot be changed once they have been created. They are thread-safe by design and can be used to ease concurrency management.
Use High-Level Concurrency Constructs
Java Concurrency API includes various high-level concurrency constructs, such as CountDownLatch, CyclicBarrier, and Semaphore, that can be used to ease concurrency management. These constructs allow you to coordinate multiple threads and ensure that they execute in a specific order.
Conclusion
- Developing multi-threaded applications is essential for improving the performance and responsiveness of applications.
- Java Concurrency API provides a wide range of tools and mechanisms for developing multi-threaded applications, including thread creation and management, synchronization, thread safety, thread pools, and executors.
- Understanding the basics of multi-threaded programming and the Java Concurrency API is crucial for developing efficient, reliable, and scalable applications.
- It is important to follow best practices, such as minimizing the use of global variables, using thread-safe collections, avoiding deadlocks, and properly managing thread synchronization.
- Atomic variables and lock objects are important mechanisms provided by Java Concurrency API to ensure thread safety and avoid concurrency issues.
- Developing multi-threaded applications with Java Concurrency API requires careful planning, testing, and debugging to ensure that the application is scalable, reliable, and efficient.
- With proper use of Java Concurrency API, developers can create applications that can handle large amounts of data, process multiple requests simultaneously, and provide a better user experience.