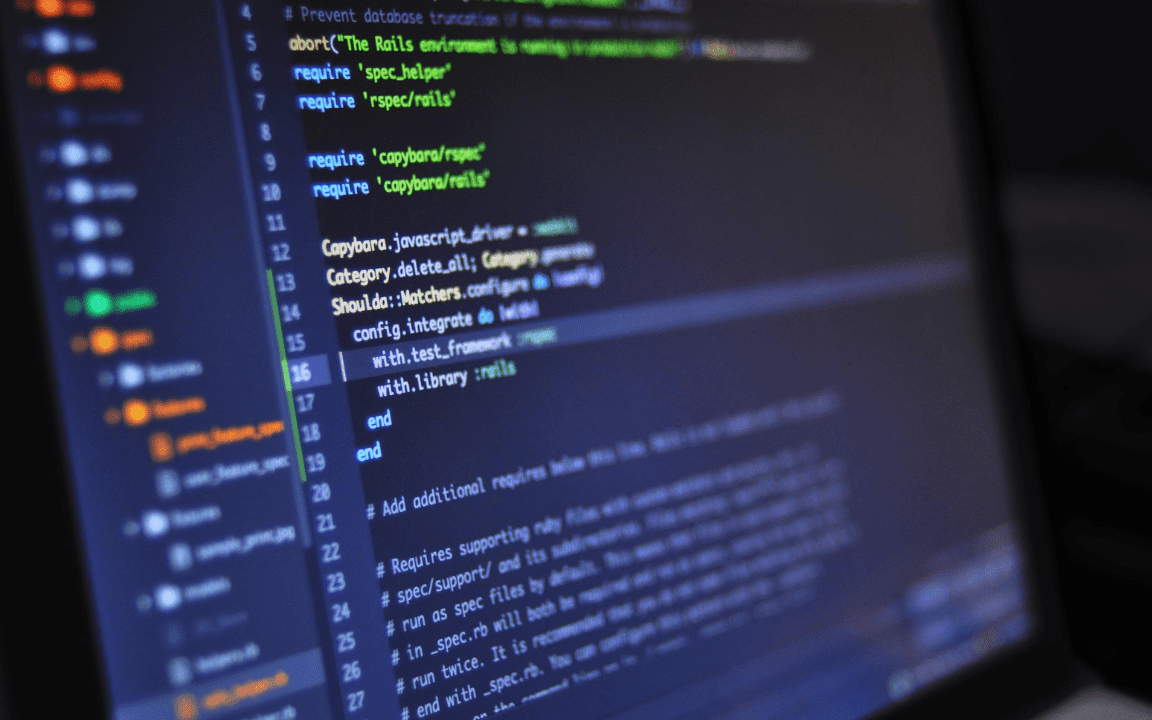
Overview
Inheritance is one of the most important pillars of Object Oriented Programming (OOP). It is the process through which one class inherits the attributes and methods of another existing class. The Parent class/superclass is the one whose attributes and methods are inherited. The Child class/subclass is the one that inherits the parent class properties.
The important point is that a child class can have its own properties and methods in addition to the inherited ones. The key to understanding Inheritance is that it allows for code re-usability. Instead of repeating the same code, we can just inherit the properties of one class into the other.
Java does not support multiple inheritances to avoid the diamond problem which causes complexity and ambiguity. But we can achieve multiple inheritances in Java through the concept of interfaces.
What is Inheritance?
Inheritance is one of the important features of OOPs (Object Oriented Programming System) through which a class inherits all of the attributes and methods of another class. The class that inherits the properties of another class is referred to as the subclass, while the class whose properties are being inherited is referred to as the superclass. In Java, we utilize the extend keyword to inherit a class.
The goal of inheritance is to simplify and reuse code. Consider an example, If the objects “car,” “bus,” and “motorcycle” are all subclasses of a vehicle, the code that applies to all of them can be combined into a vehicle superclass. Subclasses automatically inherit this code and any subsequent changes made to it.
Important terms used in inheritance are:
- Class: A class is a group of objects that share common characteristics/behaviour and common properties/attributes. Class is not a real-world entity. It is simply a template, blueprint, or prototype from which objects are constructed.
- Super Class/Parent Class: A superclass is a class whose characteristics are inherited (or a base class or a parent class).
- Subclass/Child Class: A subclass is a class that inherits from another class (or a derived class, extended class, or child class). In addition to the superclass fields and methods, the subclass can add its own.
- Reusability: The principle of reusability is highly supported by Java. Java classes can be reused in a variety of ways. Once a class has been built and tested, another programmer can modify it to meet their needs. This is accomplished primarily by creating new classes and utilizing the characteristics of existing ones. The logic behind inheritance is that we can create new classes from existing classes. When you inherit from an existing class, you can reuse the parent class’s methods and fields while also adding new methods and fields. This is how inheritance contributes to reusability.
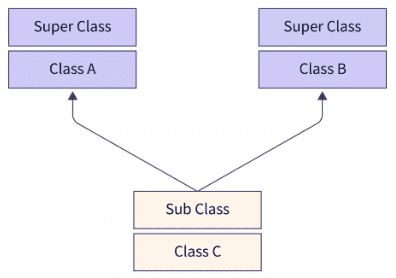
Types of Inheritance
Single Inheritance
Single Inheritance is the inheritance of a single derived class from a single base class. It is the simplest type of inheritance because it does not include any inheritance combinations or distinct levels of inheritance.
If approached correctly, single inheritance is safer than multiple inheritances. It also allows a derived class to call the parent class implementation for a certain method if that method is overridden in the derived or parent class constructors.
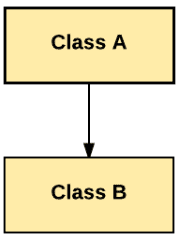
In the above image, Class B extends only to Class A. Class B is the child class that inherits the characteristics of Class A which is a parent class.
Implementation of Single inheritance in Java
class A{
void display(){System.out.println("I am method from class A");}
}
class B extends A{
void print(){System.out.println("I am method from class B");}
}
class TestInheritance{
public static void main(String args[]){
B objB = new B();
objB.display();
objB.print();
}
}
Output of the above code:

Multiple Inheritance
Multiple inheritances refer to the ability of an object or class to inherit features from more than one parent object or parent class. It differs from single inheritance, in which an object or class may only inherit from one object or class.
Multiple inheritances is not considered good as it increases complexity and ambiguity in cases such as the “diamond problem”, where it may be unclear from which parent class a particular feature is inherited when more than one parent class implements that feature.
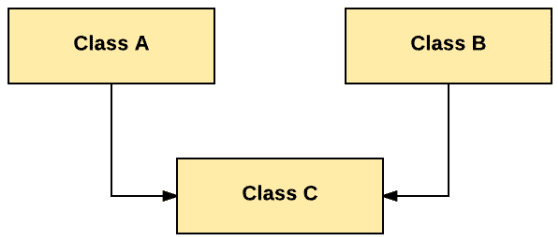
In the above image, Class C extends Class A and Class B. Class C is the child class that inherits the characteristics of Class A and Class B which are the parent classes.
Implementation of Multiple inheritances in C++
#include<iostream>
using namespace std;
class A
{
public:
A() { cout << "I am constructor from class A" << endl; }
};
class B
{
public:
B() { cout << "I am constructor from class B" << endl; }
};
class C: public B, public A // Note the order
{
public:
C() { cout << "I am constructor from class C" << endl; }
};
int main()
{
C c;
return 0;
}
The output of the above code:
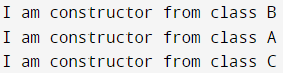
Note: Java does not support multiple inheritance. If we want to implement multiple inheritance in Java, we can use the interface concept, which also solves the ambiguity problem.
Multilevel Inheritance
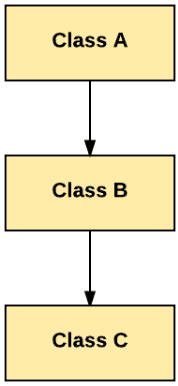
Multilevel inheritance is a type of inheritance in which there is an involvement of at least two or more subclasses. There are three class levels in Multilevel Inheritance: base class, intermediate class, and derived class.
In the above image, Class B extends Class A and Class C further extends Class B. Class B is the parent class of Class C while Class A is the parent class of Class B. As a result, the concept of grandparent class emerges in multi-level inheritance. Class C inherits the methods and properties of both Classes A and B.
Implementation of Multilevel Inheritance in Java
class A{
void display(){System.out.println("I am method from class A");}
}
class B extends A{
void print(){System.out.println("I am method from class B");}
}
class C extends B{
void react(){System.out.println("I am method from class C");}
}
class TestInheritance{
public static void main(String args[]){
C objC = new C();
objC.display();
objC.print();
objC.react();
}
}
The output of the above code:
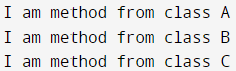
Hierarchical Inheritance
Hierarchical inheritance is the type of inheritance in which several subclasses inherit from a single class. Hierarchical inheritance is a combination of many types of inheritance.
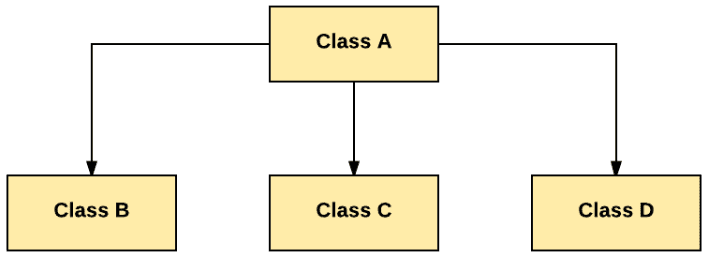
In the above image, Class B, C, and D extend Class A. Class B, C, and D are the child classes that inherit the characteristics of Class A which is a parent class. We can see that all child classes have the same parent class in hierarchical inheritance.
Implementation of Hierarchical inheritance in Java
class A{
void display(){System.out.println("I am method from class A");}
}
class B extends A{
void print(){System.out.println("I am method from class B");}
}
class C extends A{
void react(){System.out.println("I am method from class C");}
}
class D extends A{
void exact(){System.out.println("I am method from class D");}
}
class TestInheritance{
public static void main(String args[]){
B objB = new B();
objB.print();
objB.display();
C objC = new C();
objC.react();
objC.display();
D objD = new D();
objD.exact();
objD.display();
}
}
The output of the above code:
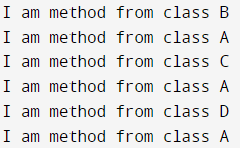
Hybrid Inheritance
The term “hybrid” inheritance indicates a combination of multiple types of inheritance. You’ve probably heard of hybrid vehicles, which can run on both gasoline and electricity. The same is true for hybrid inheritance.
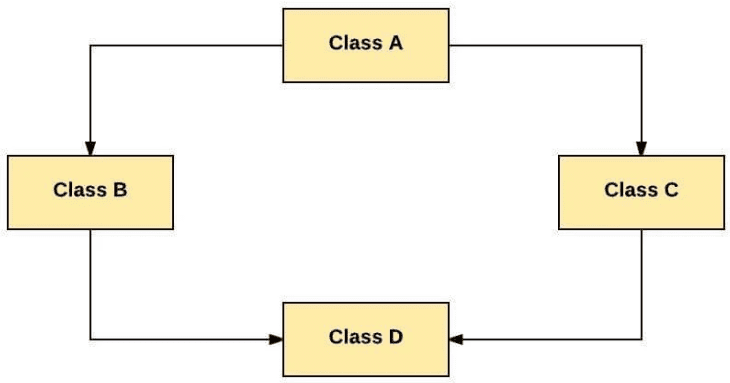
Hybrid inheritance is a combination of many types of inheritance. It can be a combination of “Single and Multiple Inheritance,” “Multilevel and Hierarchical Inheritance,” or any other combination that your software requires. The fundamental goal of Hybrid Inheritance is to make it easier for the programmer to reuse methods and properties that are already present in other classes.
In the above image, Class D extends Class B and C, while Class B and C extend Class A. Class B and C, are the child classes that inherit the characteristics of Class A while Class D is the child class that inherits the characteristics of Class B and C.
What is Inheritance in Java?
Inheritance is the process through which a class inherits all of the attributes and methods of another existing class. The class that inherits the properties of another class is referred to as the subclass, while the class whose properties are being inherited is referred to as the superclass. In Java, we use the extend keyword to inherit a class.
Syntax of Inheritance in Java
class subClass extends superClass
{
//methods and fields
}
Why Java does not Support Multiple Inheritance?
Multiple inheritance is supported by C++, Python, and a few other languages, but not by Java. To prevent the ambiguity produced by multiple inheritance, Java does not allow it. The diamond problem, which happens in multiple inheritance, is one example of such a problem.
There are two reasons given below that explain why Java does not support multiple inheritance.
- The Diamond Problem:
Consider a class A that has a foo() method and then B and C that derive from A and have their own foo() implementation and now class D that derives from B and C using multiple inheritance and the compiler will not be able to distinguish which foo() it should execute if we only refer to foo(). This is also known as the Diamond problem because the structure of this inheritance scenario is comparable to a four-edged diamond, as shown below.
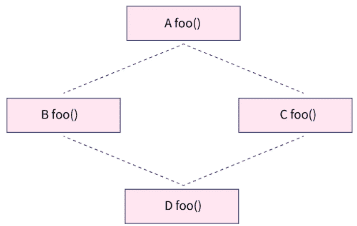
- Simplicity:
Dealing with the complexity caused by multiple inheritance is quite difficult. It causes issues during various operations like casting and constructor chaining, and all the above-mentioned reason is that there are very few circumstances in which multiple inheritance is required, thus it is preferable to avoid it to keep things simple and easy.
How to Achieve Multiple Inheritance in Java
Multiple Inheritance is a concept in object-oriented programming that allows a class to inherit properties from one or multiple parent classes. The problem arises when methods with similar signatures exist in both subclasses and superclasses. The compiler is unable to determine which class method should be called first or given priority when calling the method.
Multiple inheritance creates ambiguity. For example, assume there is a Sub class and two classes named Cat and Dog, each with a method called sample( ). And if the class Sub inherits both Cat and Dog, there will be two copies of the sampling method, one from each superclass, making it impossible to decide which method to use.
In Java, we can achieve multiple inheritance through the concept of interface. An interface is like a class that has variables and methods, however, unlike a class, the methods in an interface are abstract by default. Multiple inheritance through interface occurs in Java when a class implements multiple interfaces or when an interface extends multiple interfaces.
In this example, we’ll see how a Java program illustrates multiple inheritance via an interface.
- Each interface, Dog and Cat, has one abstract method, i.e., bark() and meow(), respectively. The Animal class implements the interfaces Dog and Cat.
- The main() method of the Demo class creates an object of the Animal class. Then, the bark() and meow() functions are called.
- Implementation of Multiple inheritance in Java through Interface
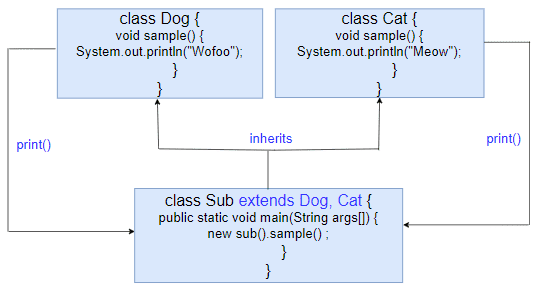
interface Dog {
void bark();
}
interface Cat {
void meow();
}
class Animal implements Dog, Cat {
public void bark() {
System.out.println("Dog is barking");
}
public void meow() {
System.out.println("Cat is meowing");
}
}
class Main {
public static void main(String args[]) {
Animal a = new Animal();
a.bark();
a.meow();
}
}
Output of the above code:
Dog is barking
Cat is meowing
Conclusion
- Inheritance is one of the important features of OOPs (Object Oriented Programming System) through which a class inherits all of the attributes and methods of another class.
- The class that inherits the properties of another class is referred to as the child class, while the class whose properties are being inherited is referred to as the parent class. In Java, we utilize the extend keyword to inherit a class.
- Single Inheritance is the inheritance of a single derived class from a single base class while in multiple inheritance a child class can inherit features from more than one parent object or parent class.
- Multiple inheritance is supported by C++, Python, and a few other languages, but not by Java. To prevent the ambiguity produced by multiple inheritance, Java does not allow it. The diamond problem, which happens in multiple inheritance, is one example of such a problem.
- We can achieve multiple inheritance in Java through the concept of interfaces.