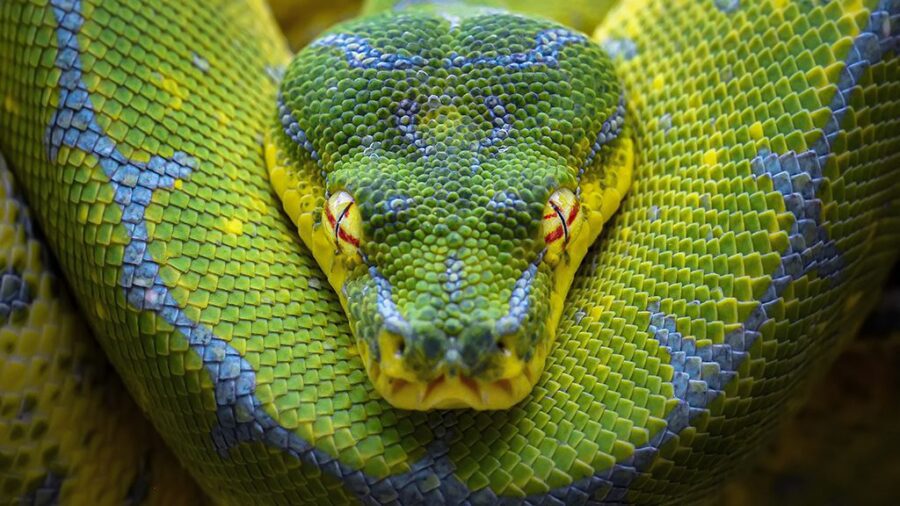
Python is an object-oriented and high-level language that supports many built-in functions which is easy to use for users.
Python has built-in functions for handling files such as creating a file, writing into the file, reading the file, and updating the file.
Scope of the Article
→ In this article, we will know about file-handling functions in python.
→ We will know each file handling function, such as opening the file, closing the file, creating a file, writing into the file, reading the file, and updating the file.
→ Some methods in file handling in python.
Introduction
→ File handling functions are creating a file, writing into the file, reading the file, and updating the file.
→ Files are generally used to store information fetched from the programs.
→ Files are of 2 types such as normal text files and binary files.
→ Text files have a terminator for each line with a special character in python.
→ Binary files are written in binary languages i.e., 0s and 1s, which does not require a terminator.
→ As these are binary files data is stored in a file after converting it into machine-understandable binary language.
→ We have different modes of accessing files. Let us discuss them.
Access Modes for using files
Access modes here are used to open a file in that particular mode and then access with the specified mode.
Access modes in python are,
- Read Only (‘r): Opening a file for only reading, and a file pointer positioned at the beginning of the file.
If the file selected does not exist, it returns an error.
- Read and Write (‘r+’): Opening a file for reading and writing, the file pointer is positioned at the beginning of the file.
If the file selected does not exist, it returns an error.
- Write Only (‘w’): Opening the file for writing content to the file, the file pointer position at the beginning of the file.
If the file selected does not exist, it returns an error.
- Write and Read (‘w+’): Opening a file for reading and writing, the file pointer is positioned at the beginning of the file.
- Append Only (‘a’): Opening the file for writing, the file pointer is positioned at the end of the file.
If the file selected does not exist, it creates a file and uses it.
- Append and Read(‘a+’): Opening the file for reading and writing, the file pointer is positioned at the end of the file.
If the file selected does not exist, it creates a file and uses it.
- Binary (‘b’): Opening the file in binary mode.
Now, Let us know the operations that can be done on files.
Opening the File in Python
Python has a built-in function for opening a file that is open() function.
Syntax:
f = open(“file name”, “Access mode”)
Example:
file = open(“C:/PythonPrograms/sample.txt”)
We can also specify the mode of the file,
Opening the file in write mode,
file = open(“C:/PythonPrograms/sample.txt”, ‘w’)
Opening the file in read mode,
file = open(“C:/PythonPrograms/sample.txt”, ‘r’)
Opening the file in read and write in binary mode,
file = open(“C:/PythonPrograms/sample.txt”, ‘r+b’)
We can also write the statement as
file = open(“C:/PythonPrograms/sample.txt”, mode= ‘r+b’)
And it is recommended to write encoding type,
file = open(“C:/PythonPrograms/sample.txt”, mode= ‘r+b’, encoding= ‘utf-8’)
Closing the file in python:
Python has a built-in function for closing a file that is close() function.
Syntax:
file.close() #file is created file name.
Example:
file = open(“C:/PythonPrograms/sample.txt”)
file.close()
Closing a file using the close() method in python.
We can also write it in a safe way using try and finally blocks,
try:
file = open(“demo.txt”, encoding = ‘utf-8’)
finally:
file.close()
This way helps us from occurring exceptions.
We also have another way to close the file using with statement as,
with open(“demo.txt”, encoding = ‘utf-8’) as f:
#executable statements
Writing to the file in Python
Python has a built-in function for writing a file which is the write() function.
Syntax:
f.write() #f is file created file name
Example:
with open(“demo.txt”, encoding = ‘utf-8’) as f:
f.write(“This is file created using python code”)
f.write(“This is next line of file”)
If demo.txt exists in the specified location, it writes the specified content in the specified file.
If demo.txt does not exist in the specified location, it shows an error.
writelines() method is used to write items in a list. The newline characters (“\n”) are part of the string.
L = [“This is a created file. \n”, This file is taken as an example. \n”]
F = open(“demo.txt”, “w”)
F.writelines(L)
F.close()
Output:
This is a created file.
This file is taken as an example.
Reading the file in Python
Python has a built-in function for Reading a file that is the read() function.
Syntax:
f.read(size to read) #f is file created file name
Example:
with open(“demo.txt”, encoding = ‘utf-8’) as f:
f.read(10) # reads the first 10 contents of the file
If demo.txt exists in the specified location, it reads the specified content in the specified file.
If demo.txt does not exist in the specified location, it shows an error.
For reading entire file write, print(f.read())
We can read a file line-wise using a for loop,
for i in f:
print(i, end= ‘ ’)
# reads content line by line.
We can read a single line using readline()
f.readline()
Used to read individual lines.
Appending to the file in Python
For appending the content to the file we open the file using the open() method in append(a) mode and then use the write() method to write content into the file.
F = open(“sample1.txt”, ‘a’)
F.write(“Appending this sentence to the file”)
F.close()
For every python program, when we use the files, the closing of the file must use the close() method.
We can also open the file using the open() method in append plus(a+) mode and then use the write() method to write content into the file.
F = open(“sample1.txt”, ‘a+’)
F.write(“Appending this sentence to the file”)
F.close()
This writes or appends the content to the file and then also reads the file and at last closes the file.
We also have some methods in file handling in python, seek() method and the tell() method.
seek() method is used to change the position of the file pointer.
tell() method is used to tell the current position of the file pointer.
Other methods such as detach(), fileno(), flush(), isatty()..
Conclusion:
1. Firstly, we discussed files and why file handling is used in python.
2. We know the types of files, normal text files, and Binary files.
3. Text files have a terminator for each line with a special character in python.
4. Binary files are written in binary languages i.e., 0s and 1s, which does not require a terminator.
5. We had known the access mode for handling the files in python, access modes are read mode(r), read plus mode(r+), write(w), write plus(w+), append(a), append plus(a+), binary mode(b) and there are many other modes in python for handling files.
6. These modes are used when we open a file and mention the mode of file to open it, it opens the file with that respective mode.
7. We had discussed each mode with examples using python programming.
8. Atlast we discussed various other methods used for file handling in python.
Hope you enjoyed the blog and learned some new knowledge from this Blog 🙂